Components
React-pdf follows the React primitives specification, making the learning process very straightforward if you come from another React environment (such as react-native). Additionally, it implements custom Component types that allow you to structure your PDF document.
Document
This component represents the PDF document itself. It must be the root of your tree element structure, and under no circumstances should it be used as child of another react-pdf component. In addition, it should only have children of type <Page />
.
Valid props
Prop name | Description | Type | Default |
---|---|---|---|
title | Sets title info on the document's metadata | String | undefined |
author | Sets author info on the document's metadata | String | undefined |
subject | Sets subject info on the document's metadata | String | undefined |
keywords | Sets keywords associated info on the document's metadata | String | undefined |
creator | Sets creator info on the document's metadata | String | "react-pdf" |
producer | Sets producer info on the document's metadata | String | "react-pdf" |
pdfVersion | Sets PDF version for generated document | String | "1.3" |
language | Sets PDF default language | String | undefined |
pageMode | Specifying how the document should be displayed when opened | PageMode | useNone |
pageLayout | This controls how (some) PDF viewers choose to show pages | PageLayout | singlePage |
onRender | Callback after document renders. Receives document blob argument in web | Function | undefined |
PageMode type
pageMode
prop can take one of the following values. Take into account some viewers might ignore this setting.
Value | Description |
---|---|
useNone | Neither document bookmarks nor thumbnail images visible |
useOutlines | Document bookmarks visible |
useThumbs | Thumbnail images visible |
fullScreen | Full-screen mode, with no menu bar, window controls, or any other window visible |
useOC | Optional content group panel visible |
useAttachments | Attachments panel visible |
PageLayout type
pageLayout
prop can take one of the following values. Take into account some viewers might ignore this setting.
Value | Description |
---|---|
singlePage | Display one page at a time |
oneColumn | Display the pages in one column |
twoColumnLeft | Display the pages in two columns, with odd numbered pages on the left |
twoColumnRight | Display the pages in two columns, with odd numbered pages on the right |
twoPageLeft | Display the pages two at a time, with odd-numbered pages on the left |
twoPageRight | Display the pages two at a time, with odd-numbered pages on the right |
Page
Represents single page inside the PDF documents, or a subset of them if using the wrapping feature. A <Document />
can contain as many pages as you want, but ensures not rendering a page inside any component besides Document.
Valid props
Prop name | Description | Type | Default |
---|---|---|---|
size | Defines page size. If String, must be one of the available page sizes. Height is optional, if ommited it will behave as "auto". | String, Array, Number, Object | "A4" |
orientation | Defines page orientation. Valid values: "portrait" or "landscape" | String | "portrait" |
wrap | Enables page wrapping for this page. See more | Boolean | true |
style | Defines page styles. See more | Object, Array | undefined |
debug | Enables debug mode on page bounding box. See more | Boolean | false |
dpi | Enables setting a custom DPI for page contents. | Number | 72 |
bookmark | Attach bookmark to element. See more | String or Bookmark | undefined |
View
The most fundamental component for building a UI and is designed to be nested inside other views and can have 0 to many children.
Valid props
Prop name | Description | Type | Default |
---|---|---|---|
wrap | Enable/disable page wrapping for element See more | Boolean | true |
style | Defines view styles. See more | Object, Array | undefined |
render | Render dynamic content based on context See more | Function | undefined |
debug | Enables debug mode on view bounding box. See more | Boolean | false |
fixed | Render component in all wrapped pages. See more | Boolean | false |
bookmark | Attach bookmark to element. See more | String or Bookmark | undefined |
Image
A React component for displaying network or local (Node only) JPG or PNG images, as well as base64 encoded image strings.
Valid props
Prop name | Description | Type | Default |
---|---|---|---|
src | Source of the image See Source object | Source object | undefined |
source | Alias of src See Source object | Source object | undefined |
style | Defines view styles. See more | Object, Array | undefined |
debug | Enables debug mode on view bounding box. See more | Boolean | false |
fixed | Renders component in all wrapped pages. See more | Boolean | false |
cache | Enables image caching between consecutive renders | Boolean | true |
bookmark | Attach bookmark to element. See more | String or Bookmark | undefined |
Source object
Defines the source of an image. Can be in any of these four valid forms:
Form type | Description | Example |
---|---|---|
String | Valid image URL or filesystem path (Node only) | www.react-pdf.org/test.jpg |
URL object | Enables to pass extra parameters on how to fetch images | { uri: valid-url, method: 'GET', headers: {}, body: '' } |
Buffer | Renders image directly from Buffer. Image format (png or jpg) will be guessed based on Buffer. | Buffer |
Data buffer | Renders buffer image via the data key. It's also recommended to provide the image format so the engine knows how to proccess it | { data: Buffer, format: 'png' \| 'jpg' } |
Function | A function that returns (can also return a promise that resolves to) any of the above formats | () => String \| Promise<String> |
Text
A React component for displaying text. Text supports nesting of other Text or Link components to create inline styling.
Valid props
Prop name | Description | Type | Default |
---|---|---|---|
wrap | Enables/disables page wrapping for element See more | Boolean | true |
render | Renders dynamic content based on context See more | Function | undefined |
style | Defines view styles. See more | Object, Array | undefined |
debug | Enables debug mode on view bounding box. See more | Boolean | false |
fixed | Renders component in all wrapped pages. See more | Boolean | false |
hyphenationCallback | Specify hyphenation callback at a text level. See hypthenation | Function | undefined |
bookmark | Attach bookmark to element. See more | String or Bookmark | undefined |
Link
A React component for displaying an hyperlink. Link’s can be nested inside a Text component, or being inside any other valid primitive.
Valid props
Prop name | Description | Type | Default |
---|---|---|---|
src | Valid URL | String | undefined |
wrap | Enable/disable page wrapping for element See more | Boolean | true |
style | Defines view styles. See more | Object, Array | undefined |
debug | Enables debug mode on view bounding box. See more | Boolean | false |
fixed | Render component in all wrapped pages. See more | Boolean | false |
bookmark | Attach bookmark to element. See more | String or Bookmark | undefined |
Note
A React component for displaying a note annotation inside the document.
Valid props
Prop name | Description | Type | Default |
---|---|---|---|
style | Defines view styles. See more | Object, Array | undefined |
children | Note string content | String | undefined |
fixed | Renders component in all wrapped pages. See more | Boolean | false |
Canvas
A React component for freely drawing any content on the page.
Valid props
Prop name | Description | Type | Default |
---|---|---|---|
style | Defines view styles. See more | Object, Array | undefined |
paint | Painter function | Function | undefined |
debug | Enables debug mode on view bounding box. See more | Boolean | false |
fixed | Renders component in all wrapped pages. See more | Boolean | false |
bookmark | Attach bookmark to element. See more | String or Bookmark | undefined |
React-pdf does not check how much space your drawing takes, so make sure you always define a width
and height
on the style
prop.
Painter function
Prop used to perform drawings inside the Canvas. It takes 3 arguments:
Painter object
: Wrapper around pdfkit drawing methods. Use this to draw inside the CanvasavailableWidth
: Width of the Canvas element.availableHeight
: Height of the Canvas element.
Painter object
Wrapper around pdfkit methods you can use to draw inside the Canvas. All operations are chainable. For more information about how these methods work, please refer to pdfkit documentation.
Available methods:
- dash
- clip
- save
- path
- fill
- font
- text
- rect
- scale
- moveTo
- lineTo
- stroke
- rotate
- circle
- lineCap
- opacity
- ellipse
- polygon
- restore
- lineJoin
- fontSize
- fillColor
- lineWidth
- translate
- miterLimit
- strokeColor
- fillOpacity
- roundedRect
- strokeOpacity
- bezierCurveTo
- quadraticCurveTo
- linearGradient
- radialGradient
PDFViewer Web only
Iframe PDF viewer for client-side generated documents.
Valid props
Prop name | Description | Type | Default |
---|---|---|---|
style | Defines iframe styles | Object, Array | undefined |
className | Defines iframe class name | String | undefined |
children | PDF document implementation | Document | undefined |
width | Width of embedded PDF iframe | String, Number | undefined |
height | Height of embedded PDF iframe | String, Number | undefined |
showToolbar | Render the toolbar. Supported on Chrome, Edge and Safari | Boolean | true |
Other props are passed through to the iframe.
PDFDownloadLink Web only
Anchor tag to enable generate and download PDF documents on the fly. Refer to on the fly rendering for more information.
Valid props
Prop name | Description | Type | Default |
---|---|---|---|
document | PDF document implementation | Document | undefined |
fileName | Download PDF file name | String | undefined |
style | Defines anchor tag styles | Object, Array | undefined |
className | Defines anchor tag class name | String | undefined |
children | Anchor tag content | DOM node, Function | undefined |
BlobProvider Web only
Easy and declarative way of getting document's blob data without showing it on screen. Refer to on the fly rendering for more information.
Valid props
Prop name | Description | Type | Default |
---|---|---|---|
document | PDF document implementation | Document | undefined |
children | Render prop with blob, url, error and loading state as arguments | Function | undefined |
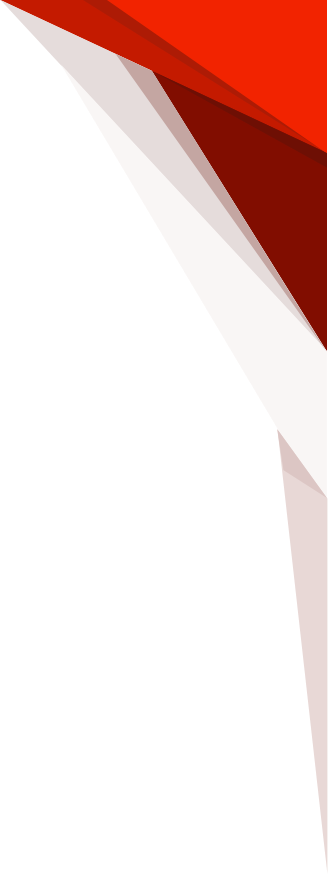